Preparing your Predictive Calculator
Enter your email to continue
Welcome, New User
Verify your email address
Welcome back
Verify your email address
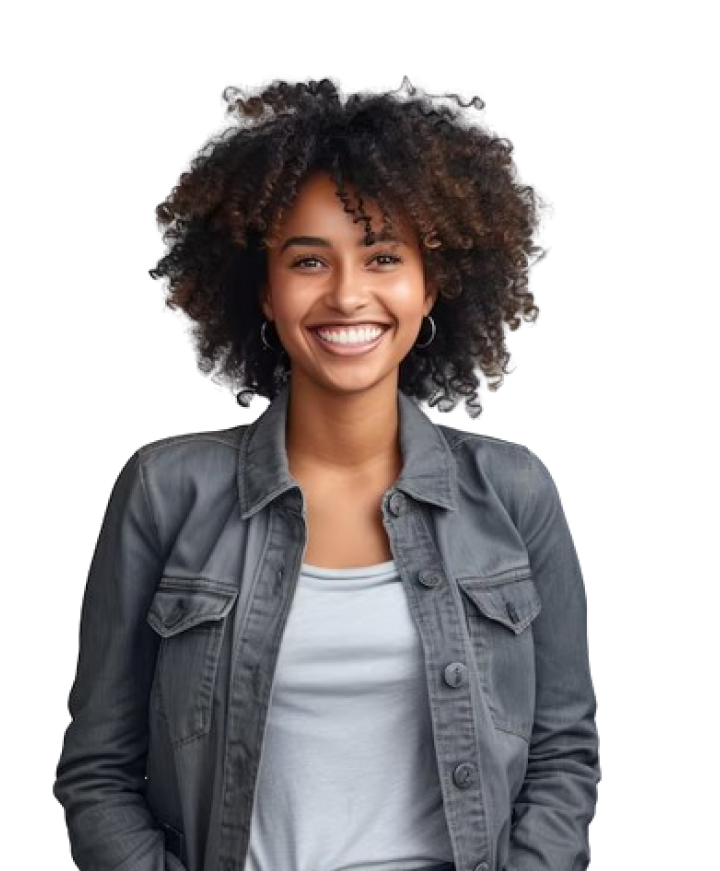
“Shirah has been an amazing partner on my journey of moving from Nigeria to Canada for studies. Honestly, I wasn’t sure how to get started with anything but after I went through Shirah’s Predictive Calculator, everything was clearer.”
How old are you?
How many years of work experience do you have?
Select which educational level best describes you.
What field is your occupation?
Are you fluent in both English and French enough to write and pass the language tests for both?
check_circle Permanent Residency
You selected Permanent Residency via Express Entry as your end goal
check_circle How old are you?
You are less than 30 years old
You are 30 years old or above
check_circle Years of experience
You have less than 3 years of work experience
You have 3 or more years of work experience
check_circle Educational level
Bachelor's Degree
Bachelor's Degree and One year Diploma/certificate
Masters Degree or PhD
Diploma and Postgraduate Diploma
Others eg Diploma (1 or 2 years)
check_circle Occupation field
French-language proficiency
Healthcare occupations
Science, Technology, Engineering and Math (STEM) occupations
Trade occupations
Transport occupations
Agriculture and Agri-food occupations
Others
check_circle Language proficiency
You are fluent in both English and French
You are not fluent in both English and French